Amazon Web Services (AWS) Premier Partner
Partnership
The cloud: an essential part of any modern enterprise. It powers agility, scalability and speed to market. But making the transition is not as simple as flipping a switch. With a commitment to pragmatic and lean processes, we bring proven experience, customer obsession and future-focused best practices to help you realize your vision.
.webp?iar=0&rev=2e59b6e899c94dd9abf81bb688cab46a&hash=1067766B105206CCC881D4ED4C3D9E7E)
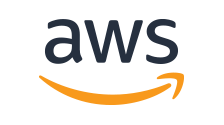
Our approach
As an AWS Premier Tier Services Partner, our deep expertise and capabilities in Amazon Web Services (AWS) allow us to meet our customers at any point in their cloud journey, whether just starting or executing on enterprise-wide transformation. Our technology partnerships take a customer-first approach by understanding your business goals and priorities, working side by side to jumpstart your transformation and maximize returns on your technology investments—from advisory, design, migration and implementation to adoption and innovation.
As an AWS Training Partner, our role-based and specialized training services are designed to empower employees, drive adoption and build effective, innovative teams for cloud initiatives using AWS.
COMPETENCIES
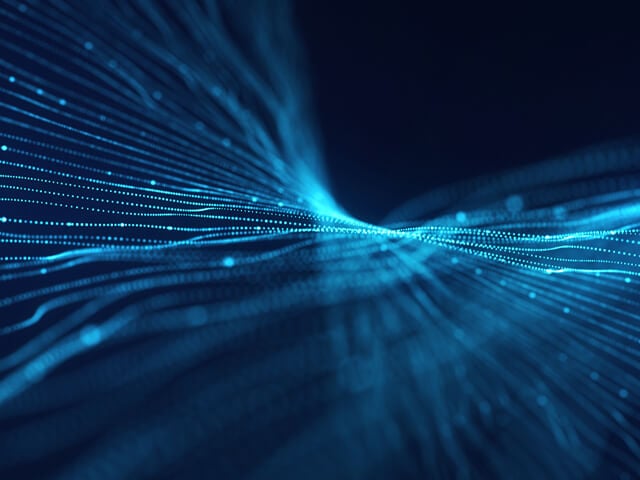
Migration
Take the first step towards modernization with cloud enablement and migration. Whether migrating specific workloads or entire data centers, our scalable and comprehensive approach builds a strong foundation for your cloud infrastructure and reduces your legacy footprint. From well-architected solutions and cloud journey maps to risk mitigation, we’ll build AWS partner solutions aligned to your business and IT goals that enable a scalable tomorrow.
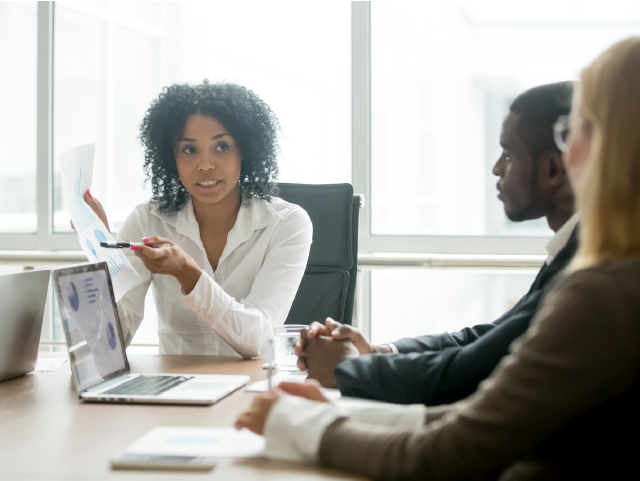
Data and Analytics
Do more, extract insights and drive value with data and analytics—from designing product roadmaps to creating better customer experiences. Level up your processing power with a modern data platform to power solutions in your industry. Break down silos for more agility, better visibility and smarter business decisions.
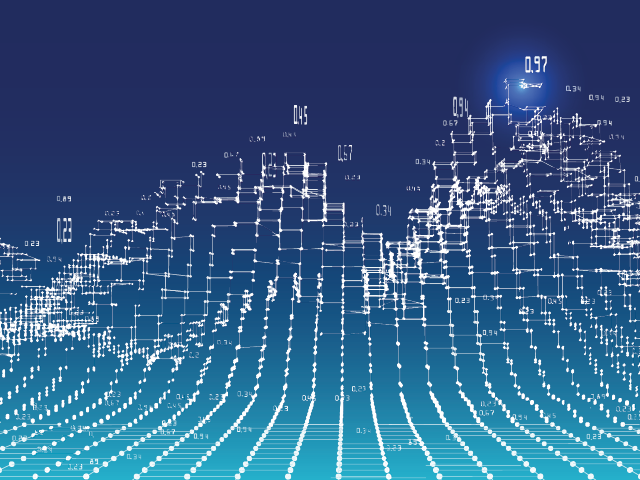
Machine Learning
Leverage our machine learning expertise to proactively drive your organization forward. Unlock new revenue streams, create frictionless customer experiences and automate processes. As an AWS premier tier services partner, we help customers use actionable insights with effective ML models to seize market opportunities.
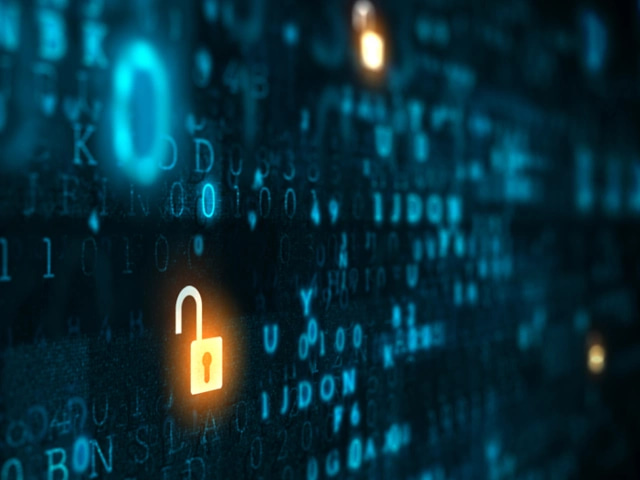
Security
From well-architected framework reviews to maturing cloud security for large enterprises, we work with you to meet the most stringent security and compliance requirements while reducing risk and minimizing potential exposure. As an AWS premier partner, we’re well-positioned to help you with security advisory, risk and control frameworks, compliant environments, review and remediation.
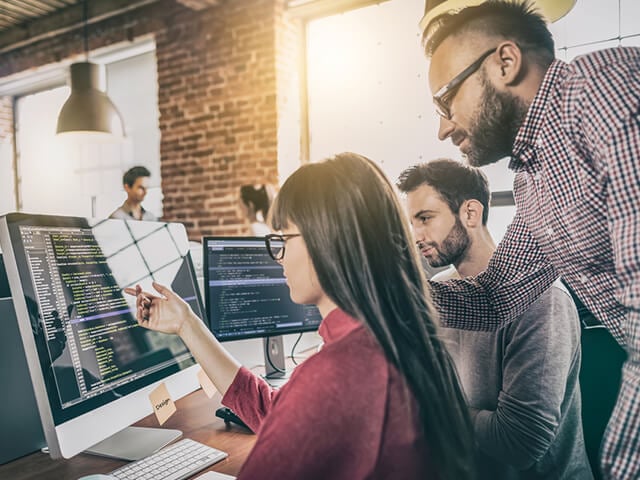
DevOps
Our DevOps services enable full-scale change by uniting development and infrastructure teams to maximize efficiency and accelerate speed to market. As an AWS premier partner, we also offer AWS-based CI/CD that spans the full-technology life cycle—from value stream mapping workshops to governance and enterprise adoption.
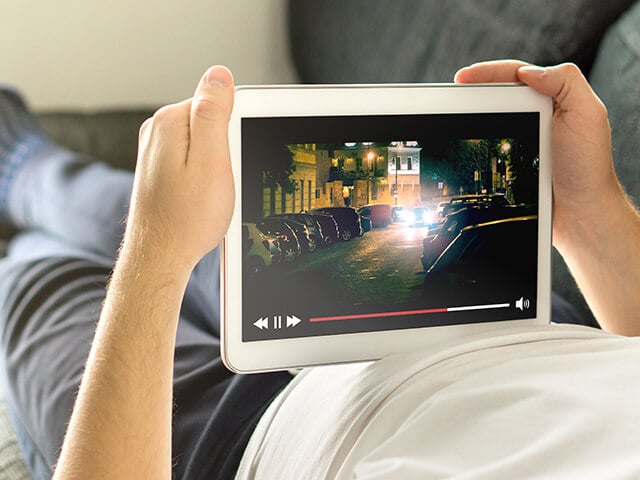
Media and Entertainment
Unlock new monetization opportunities, create efficiencies and recognize cost savings by accelerating deployments with AWS solutions in media supply chain and archive, broadcast, direct-to-consumer and streaming, and data science and analytics for media.
Discover the Power of a Consultation
Get the most from the AWS Cloud with an AWS Well-Architected Framework Review or Cloud Foundations consultation with an AWS premier partner.
Service Delivery
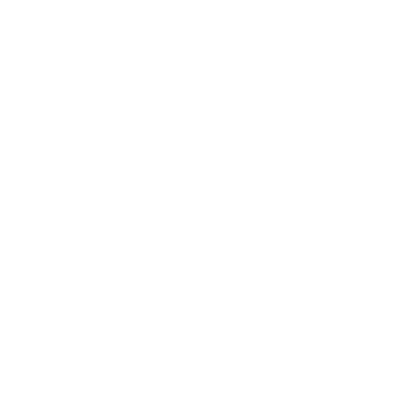
Amazon Redshift
Advisory engagements, migration, outcome-based delivery, capacity-based offerings
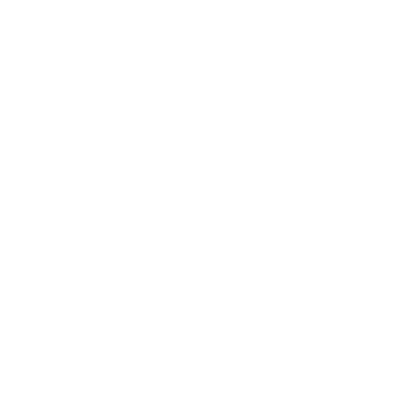
Amazon EMR
Design, build and operate elastic data platforms and smart data applications
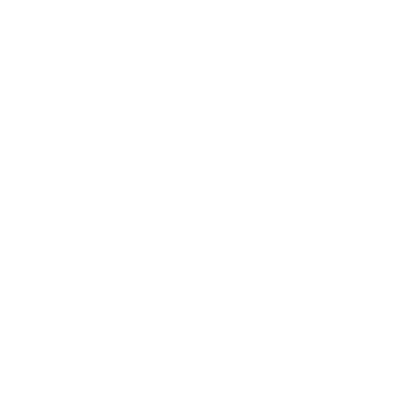
AWS Glue
Streamline modernization and legacy ETL workload migration while adding elasticity to enterprise data integration
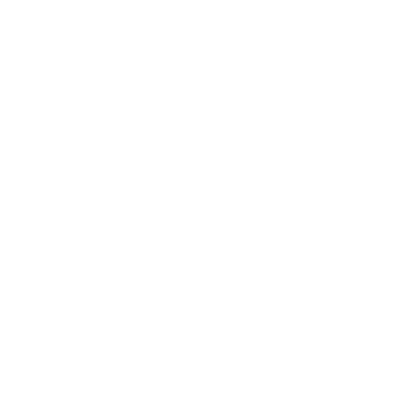
AWS Lambda
Lower total cost of ownership by going serverless for building applications
Programs
AWS Well-Architected Partner
Build secure, high-performing, resilient and efficient infrastructure that’ll mitigate risk, improve ROI and increase your scalability
APN Immersion Days
Immersive workshops give you a deep dive to test AWS services and solutions
AWS Public Sector Partner
Solutions and experience in helping government, education and nonprofit organizations achieve their missions
ProServe Ready Program
Maximize results by leveraging our proven experience working in lockstep with AWS Professional Services